Summary: in this tutorial, you’ll learn how to use the PHP substr()
function to extract a substring from a string.
Introduction to the PHP substr() function #
The substr()
function accepts a string and returns a substring from the string.
Here’s the syntax of the substr()
function:
substr ( string $string , int $offset , int|null $length = null ) : string
Code language: PHP (php)
In this syntax:
$string
is the input string.$offset
is the position at which the function begins to extract the substring.$length
is the number of characters to include in the substring. If you omit the$length
argument, thesubstr()
function will extract a substring from the$offset
to the end of the$string
. If the$length
is 0, false, or null, thesubstr()
function returns an empty string.
PHP substr() function examples #
Let’s take some examples of using the substr()
function.
1) Simple PHP substr() function example #
The following example uses the substr()
function to extract the first three characters from a string:
<?php
$s = 'PHP substring';
$result = substr($s, 0, 3);
echo $result;// PHP
Code language: PHP (php)
In this example, the substr()
function extract the first 3 characters from the 'PHP substring'
string starting at the index 0.
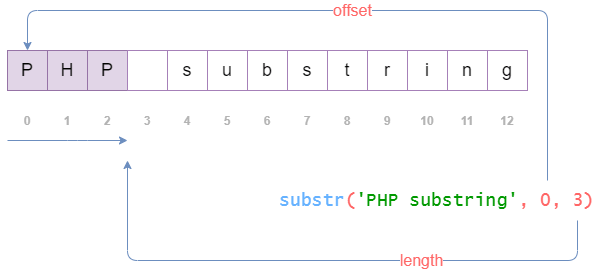
2) Using PHP substr() function with the default length argument #
The following example uses the substr()
function to extract a substring from the 'PHP substring'
string starting from the index 4 to the end of the string:
<?php
$s = 'PHP substring';
$result = substr($s, 4);
echo $result; // substring
Code language: PHP (php)
In this example, we omit the $length
argument. Therefore, the substr()
returns a substring, starting at index 4 to the end of the input string.
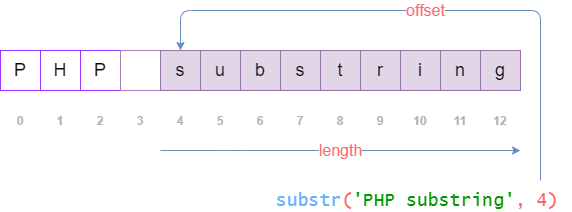
PHP substr() function with negative offset #
The $offset
argument can be a negative number. If the $offset
is negative, the substr()
function returns a substring that starts at the offset character from the end of the string. The last character in the input string has an index of -1
.
The following example illustrates how to use the substr()
function with negative offset:
<?php
$s = 'PHP is cool';
$result = substr($s, -4);
echo $result; // cool
Code language: PHP (php)
In this example, the substr()
returns a substring that at 4th character from the end of the string.
The following picture illustrates how the substr()
function works in the above example:
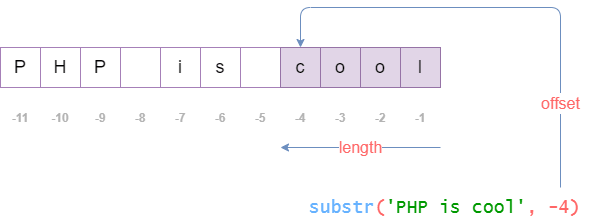
PHP substr() function with negative length #
Like the $offset
argument, the $length
argument can be negative. If you pass a negative number to the $length
argument, the substr()
function will omit a $length
number of characters in the returned substring.
The following example illustrates how to use the substr()
with a negative $offset
and $length
arguments:
<?php
$s = 'PHP is cool';
$result = substr($s, -7, -5);
echo $result; // is
Code language: PHP (php)
The following picture illustrates how the above example works:
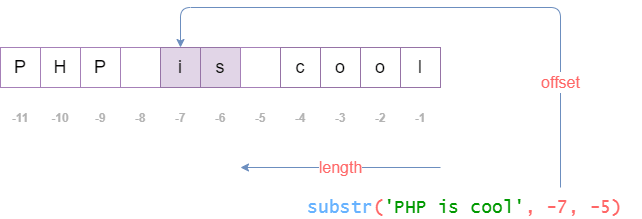
The PHP mb_substr() function #
See the following example:
<?php
$message = 'adiós';
$result = substr($message, 3, 1);
echo $result;
Code language: PHP (php)
This example attempts to extract a substring with one character in the $message
string starting at index 3. However, it shows nothing in the output.
The reason is that the $message
string contains a non-ASCII character. Therefore, the substr()
function doesn’t work correctly.
To extract a substring from a string that contains a non-ASCII character, you use the mb_substr()
function. The mb_substr()
function is like the substr()
function except that it has an additional encoding argument:
mb_substr ( string $string , int $start , int|null $length = null , string|null $encoding = null ) : string
Code language: PHP (php)
The following example uses the mb_substr()
function to extract a substring from a string with non-ASCII code:
<?php
$message = 'adiós';
$result = mb_substr($message, 3, 1);
echo $result;
Code language: PHP (php)
Output:
ó
Code language: PHP (php)
PHP substr helper function #
The following defines a helper function that uses the mb_substr()
function to extract a substring from a string:
<?php
function substring($string, $start, $length = null)
{
return mb_substr($string, $start, $length, 'UTF-8');
}
Code language: PHP (php)
Summary #
- Use the PHP
substr()
function to extract a substring from a string. - Use the negative offset to extract a substring from the end of the string. The last character in the input string has an index of
-1
. - Use the negative length to omit a length number of characters in the returned substring.
- Use the PHP
mb_substr()
function to extract a substring from a string with non-ASCII characters.