Summary: in this tutorial, you’ll learn about the PHP if statement and how to use it to execute a code block conditionally.
Introduction to the PHP if statement #
The if
statement allows you to execute a statement if an expression evaluates to true
. The following shows the syntax of the if
statement:
<?php
if ( expression )
statement;
Code language: PHP (php)
In this syntax, PHP evaluates the expression
first. If the expression
evaluates to true
, PHP executes the statement
. In case the expression evaluates to false
, PHP ignores the statement
.
The following flowchart illustrates how the if
statement works:
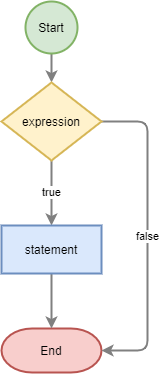
The following example uses the if
statement to display a message if the $is_admin
variable sets to true
:
<?php
$is_admin = true;
if ($is_admin)
echo 'Welcome, admin!';
Code language: PHP (php)
Since $is_admin
is true
, the script outputs the following message:
Welcome, admin!
Code language: PHP (php)
Curly braces #
If you want to execute multiple statements in the if
block, you can use curly braces to group multiple statements like this:
<?php
if ( expression ) {
statement1;
statement2;
// more statement
}
Code language: PHP (php)
The following example uses the if
statement that executes multiple statements:
<?php
$can_edit = false;
$is_admin = true;
if ( $is_admin ) {
echo 'Welcome, admin!';
$can_edit = true;
}
Code language: PHP (php)
In this example, the if
statement displays a message and sets the $can_edit
variable to true
if the $is_admin
variable is true
.
It’s a good practice to always use curly braces with the if
statement even though it has a single statement to execute like this:
<?php
if ( expression ) {
statement;
}
Code language: PHP (php)
In addition, you can use spaces between the expression and curly braces to make the code more readable.
Nesting if statements #
It’s possible to nest an if
statement inside another if
statement as follows:
<?php
if ( expression1 ) {
// do something
if( expression2 ) {
// do other things
}
}
Code language: PHP (php)
The following example shows how to nest an if
statement in another if
statement:
<?php
$is_admin = true;
$can_approve = true;
if ($is_admin) {
echo 'Welcome, admin!';
if ($can_approve) {
echo 'Please approve the pending items';
}
}
Code language: PHP (php)
Embed if statement in HTML #
To embed an if
statement in an HTML document, you can use the above syntax. However, PHP provides a better syntax that allows you to mix the if statement with HTML nicely:
<?php if ( expession) : ?>
<!-- HTML code here -->
<?php endif; ?>
Code language: PHP (php)
The following example uses the if
statement that shows the edit link if the $is_admin
is true
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>PHP if Statement Demo</title>
</head>
<body>
<?php $is_admin = true; ?>
<?php if ( $is_admin ) : ?>
<a href="#">Edit</a>
<?php endif; ?>
<a href="#">View</a>
</body>
</html>
Code language: PHP (php)
Since the $is_admin
is true
, the script shows the Edit link. If you change the value of the $is_admin
to false
, you won’t see the Edit link in the output.
A common mistake with the PHP if statement #
A common mistake that you may make is to use the wrong operator in the if
statement. For example:
<?php
$checked = 'on';
if( $checked = 'off' ) {
echo 'The checkbox has not been checked';
}
Code language: PHP (php)
This script shows a message if the $checked
is 'off'
. However, the expression in the if
statement is an assignment, not a comparison:
$checked = 'off'
Code language: PHP (php)
This expression assigns the literal string 'off'
to the $checked
variable and returns that variable. It doesn’t compare the value of the $checked
variable with the 'off'
value. Therefore, the expression always evaluates to true
, which is not correct.
To avoid this error, you can place the value first before the comparison operator and the variable after the comparison operator like this:
<?php
$checked = 'on';
if('off' == $checked ) {
echo 'The checkbox has not been checked';
}
Code language: PHP (php)
If you accidentally use the assignment operator (=), PHP will raise a syntax error instead:
<?php
$checked = 'on';
if ('off' = $checked) {
echo 'The checkbox has not been checked';
}
Code language: PHP (php)
Error:
Parse error: syntax error, unexpected '=' ...
Code language: PHP (php)
Summary #
- The
if
statement executes a statementif
a condition evaluates totrue
. - Always use curly braces even if you have a single statement to execute in the
if
statement. It makes the code more obvious. - Use the pattern
if ( value == $variable_name ) {}
to avoid possible mistakes.