Summary: in this tutorial, you will learn about PHP for
statement to execute a block of code repeatedly.
Introduction to PHP for statement
The for
statement allows you to execute a code block repeatedly. The syntax of the for
statement is as follows:
<?php
for (start; condition; increment) {
statement;
}
Code language: HTML, XML (xml)
How it works.
- The
start
is evaluated once when the loop starts. - The
condition
is evaluated once in each iteration. If thecondition
istrue
, thestatement
in the body is executed. Otherwise, the loop ends. - The
increment
expression is evaluated once after each iteration.
PHP allows you to specify multiple expressions in the start
, condition
, and increment
of the for
statement.
In addition, you can leave the start
, condition
, and increment
empty, indicating that PHP should do nothing for that phase.
The following flowchart illustrates how the for
statement works:
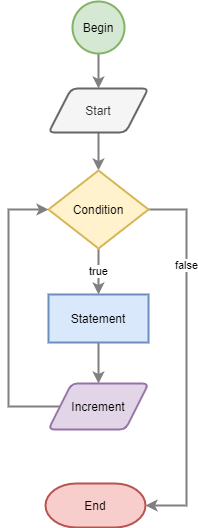
When you leave all three parts empty, you should use a break
statement to exit the loop at some point. Otherwise, you’ll have an infinite loop:
<?php
for (; ;) {
// do something
// ...
// exit the loop
if (condition) {
break;
}
}
Code language: HTML, XML (xml)
PHP for statement example
The following shows a simple example that adds numbers from 1 to 10:
<?php
$total = 0;
for ($i = 1; $i <= 10; $i++) {
$total += $i;
}
echo $total;
Code language: HTML, XML (xml)
Output:
55
How it works.
- First, initialize the
$total
to zero. - Second, start the loop by setting the variable
$i
to 1. This initialization will be evaluated once when the loop starts. - Third, the loop continues as long as
$i
is less than or equal to10
. The expression$i <= 10
is evaluated once after every iteration. - Fourth, the expression
$i++
is evaluated after each iteration. - Finally, the loop runs exactly
10
iterations and stops once$i
becomes11
.
Alternative syntax of the for statement
The for statement has the alternative syntax as follows:
for (start; condition; increment):
statement;
endfor;
Code language: PHP (php)
The following script uses the alternative syntax to calculate the sum of 10 numbers from 1 to 10:
<?php
$total = 0;
for ($i = 1; $i <= 10; $i++):
$total += $i;
endfor;
echo $total;
Code language: HTML, XML (xml)
Output:
55
Summary
- Use the PHP
for
statement to execute a code block in a specified number of times.